对象缓存
所谓对象缓存,指把id映射为实体对象。这是最简单最基础的缓存
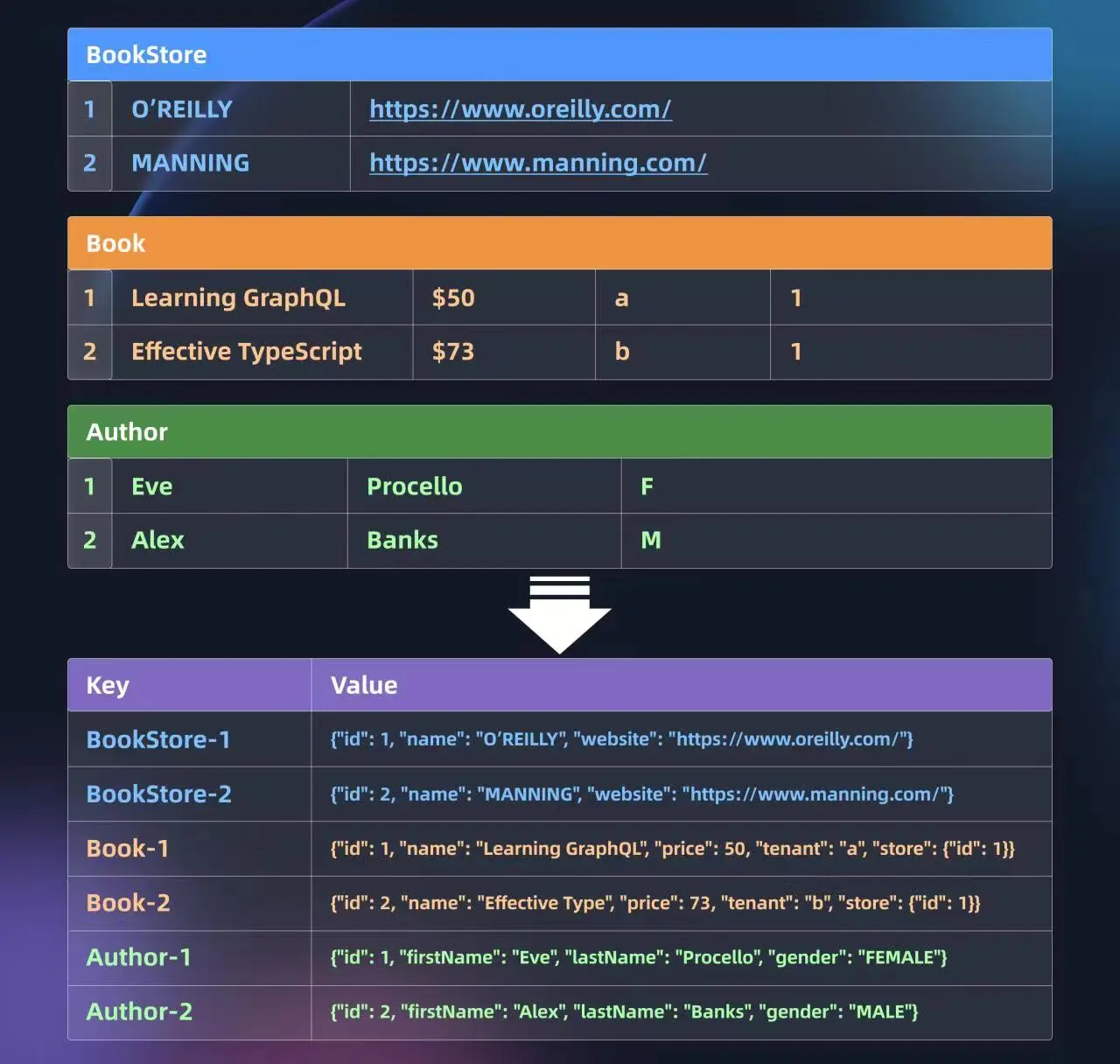
启用对象缓存
- Java
- Kotlin
@Bean
public CacheFactory cacheFactory(
RedisConnectionFactory connectionFactory,
ObjectMapper objectMapper
) {
return new CacheFactory() {
@Override
public Cache<?, ?> createObjectCache(@NotNull ImmutableType type) {
return new ChainCacheBuilder<>()
.add(
CaffeineValueBinder
.forObject(type)
.maximumSize(1024)
.duration(Duration.ofHours(1))
.build()
)
.add(
RedisValueBinder
.forObject(type)
.redis(connectionFactory)
.objectMapper(objectMapper)
.duration(Duration.ofHours(24))
.build()
)
.build();
}
...省略其他代码...
};
}
@Bean
fun cacheFactory(
connectionFactory: RedisConnectionFactory,
objectMapper: ObjectMapper
): KCacheFactory {
return object: KCacheFactory {
override fun createObjectCache(type: ImmutableType): Cache<*, *>? =
ChainCacheBuilder<Any, Any>()
.add(
CaffeineValueBinder
.forObject(type)
.maximumSize(1024)
.duration(Duration.ofHours(1))
.build()
)
.add(
RedisValueBinder
.forObject(type)
.redis(connectionFactory)
.objectMapper(objectMapper)
.duration(Duration.ofHours(24))
.build()
)
.build()
...省略其他代码...
}
}
如果不想为某些实体类型支持缓存,返回null即可
- Java
- Kotlin
@Override
public Cache<?, ?> createObjectCache(ImmutableType type) {
if (type.getJavaClass() == SomeEntity.class) {
return null;
}
...
}
override fun createObjectCache(type: ImmutableType): Cache<*, *>? =
if (type.javaClass === SomeEntity::class.java) {
null
} else {
...
}